Testing is crucial in software development. Pytest and Unittest are popular tools for Python testing.
Both have unique features and benefits. Comparing Pytest and Unittest helps developers choose the best tool for their needs. Pytest, known for its simplicity, allows writing fewer lines of code. Unittest, a built-in module, offers robustness and integration. Deciding between them can impact your workflow and project efficiency.
In this post, we’ll explore their core features, advantages, and differences. This will help you make an informed decision. Stay tuned to find out which testing tool suits your project best.
Pytest Overview
When you’re delving into the world of Python testing frameworks, Pytest stands out with its simplicity and power. If you’ve ever felt overwhelmed by the verbosity of Unittest, Pytest might just be your breath of fresh air. Let’s explore what makes Pytest a favorite among developers.
Key Features
Pytest is praised for its straightforward syntax. You don’t have to write complex test classes—just simple functions will do. This makes your tests easier to read and write.
The framework supports fixtures, which allow you to set up initial conditions for your tests. Imagine having a clean slate every time you run your tests—that’s the magic of fixtures.
Another standout feature is its powerful assertion system. You can write expressive assertions that give clear feedback on test failures, saving you from cryptic error messages.
Advantages
Pytest is flexible and can handle a variety of testing needs, from simple unit tests to complex functional tests. You’re not boxed into a rigid structure.
It’s compatible with other testing libraries. So, if you have existing Unittest code, Pytest can run those tests too, facilitating a smooth transition.
Pytest’s extensive plugin architecture lets you extend its functionality. Have you ever wanted to customize your testing process? With Pytest, you can tailor it to your needs.
Pytest isn’t just a tool—it’s a community. You’ll find active discussions, numerous tutorials, and plugins that cater to niche requirements. Have you ever been part of a thriving community that not only supports your work but enhances it? Pytest offers just that.
As you navigate your testing journey, ask yourself: What tools genuinely simplify your work? Pytest’s simplicity might be your answer. Consider trying it in your next project and discover how it can streamline your testing workflow.
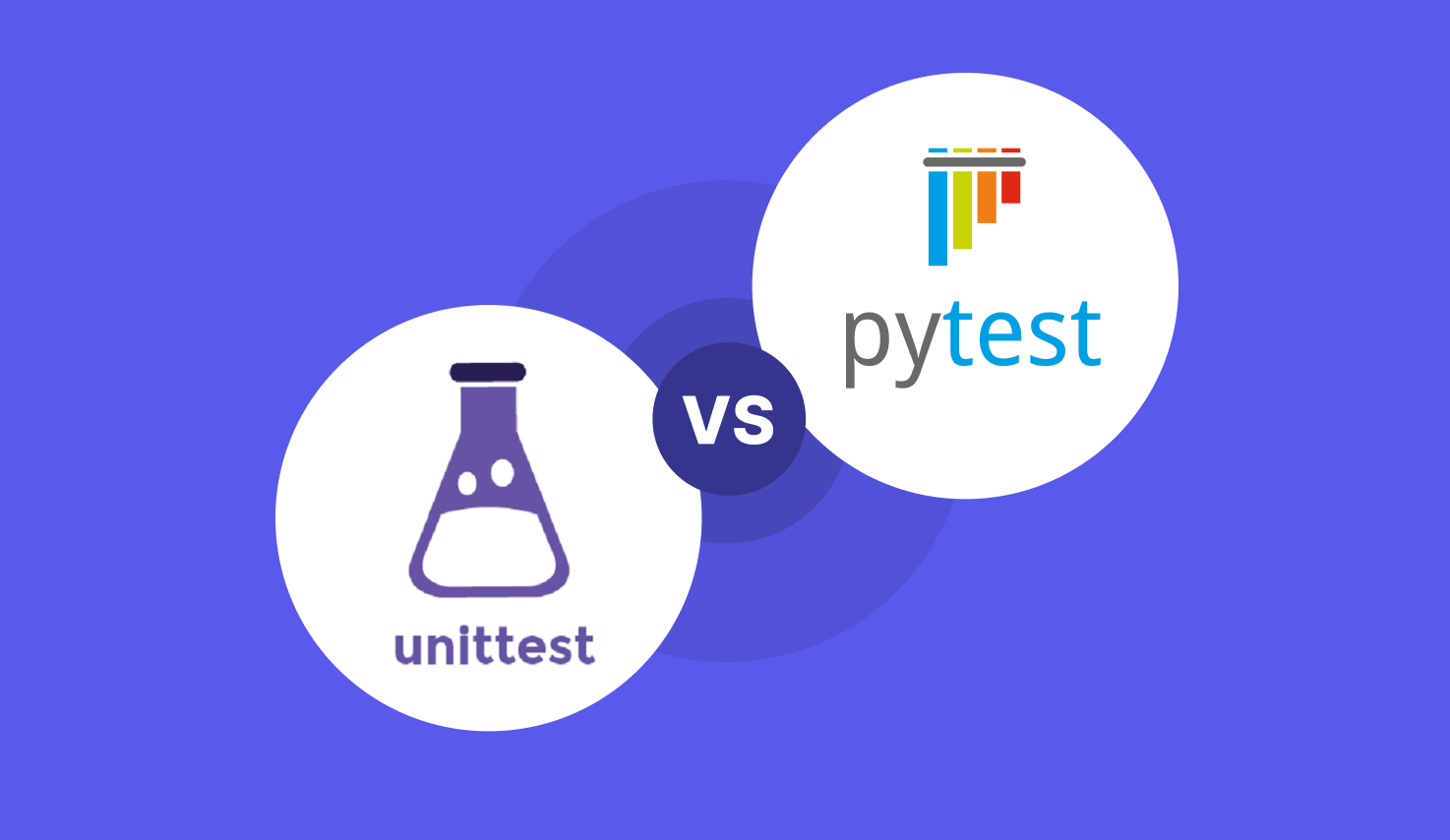
Credit: katalon.com
Unittest Overview
Unittest is a built-in testing framework in Python. It allows developers to write test cases for their code. These tests help ensure that the code works as expected. Unittest is part of Python’s standard library. This makes it easy to use without extra installations. It follows the xUnit style, popular in many programming languages.
Key Features
Unittest provides several essential features for testing. It supports test automation. This means tests can run without manual intervention. Unittest allows test aggregation. Tests can be grouped and run together. It offers rich assertions. These help check if conditions are met. Test fixtures are another feature. They prepare test environments and clean up afterward. Unittest also provides test discovery. This feature finds tests in a directory.
Advantages
Unittest is simple to learn and use. It’s part of Python’s standard library, so no extra setup is needed. Unittest works well for small projects. It ensures code runs correctly. The framework is stable and widely used. Many developers are familiar with it. Unittest has good documentation. This helps developers understand how to use it. It integrates well with other tools. This makes it versatile for different projects.
Ease Of Use
When choosing between Pytest and Unittest, ease of use is a crucial factor to consider. Both frameworks have their own strengths and weaknesses. Understanding which one is more user-friendly can help you decide which to use for your projects.
Setup And Configuration
Setting up Pytest is straightforward. You can install it using a simple pip command: pip install pytest
. There’s no need for boilerplate code, which saves time and keeps your test files clean.
Unittest, on the other hand, is part of the Python standard library. This means no additional installations are necessary. However, it does require more boilerplate code for setup, which can be cumbersome, especially for beginners.
Consider this: Do you prefer a quick setup with minimal code, or do you value the reliability of a built-in library despite the extra setup? Your answer will guide your choice.
Learning Curve
Pytest is known for its simplicity and readability. Its syntax is intuitive, making it easy for beginners to write their first tests. Functions are used instead of classes, which feels more natural to many developers.
Unittest follows a more traditional, class-based approach. This can be more challenging for those new to testing. However, if you are familiar with object-oriented programming, you might find this structure comfortable.
Think about your learning style. Do you prefer a more straightforward, less structured approach, or do you thrive on structure and familiarity with object-oriented concepts?
In my experience, starting with Pytest made the transition to testing smoother. Its ease of use allowed me to focus on writing effective tests rather than getting bogged down by setup details. But remember, your experience may differ, and the best tool is the one that fits your workflow.
What are your thoughts? Have you tried both frameworks? Which one did you find easier to use?
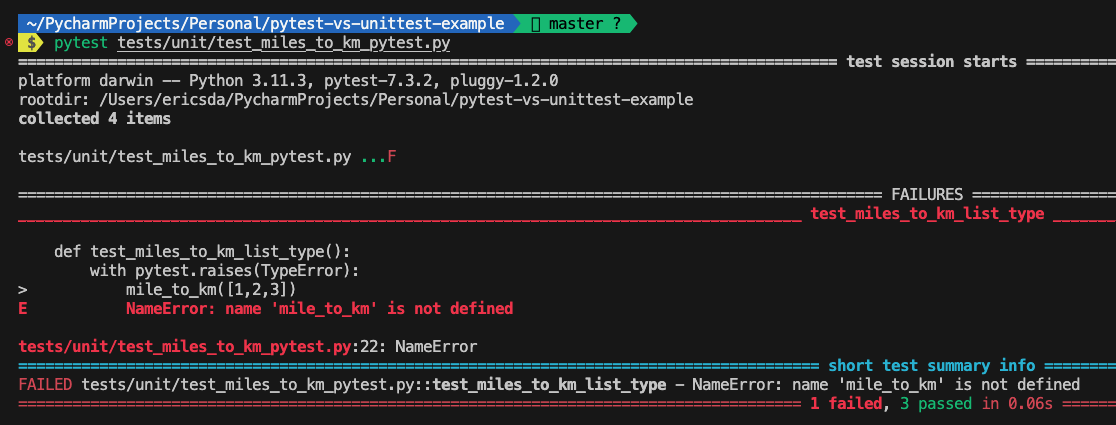
Credit: pytest-with-eric.com
Functionality
In the realm of Python testing, two popular frameworks stand out: Pytest and Unittest. Both offer unique functionality that caters to different needs. Understanding their key features helps in selecting the right tool for testing needs.
Test Discovery
Pytest excels in test discovery. It automatically identifies test files and functions. This feature saves time and effort. Test names start with “test_” for easy recognition. Pytest discovers tests without extra configuration.
Unittest relies on a more structured approach. Test cases must be organized in classes. Each test method should start with “test”. This method is more traditional. It requires a clear structure and naming convention.
Fixtures
Fixtures in Pytest simplify test setup. They allow code reuse across tests. Define fixtures using decorators. These fixtures provide resources for tests. Pytest fixtures are flexible and powerful.
Unittest uses setup and teardown methods. These methods prepare the test environment. They run before and after each test. This approach requires more boilerplate code. It is less flexible than Pytest’s fixtures.
Performance
Performance plays a critical role in choosing a testing framework. Pytest and Unittest are popular choices among developers. Both frameworks offer unique advantages. Understanding their performance differences can guide your choice. Let’s explore their performance aspects.
Speed Of Execution
Pytest often executes tests faster. It uses fixtures and plugins that streamline testing. This reduces the time needed to run tests. Its ability to run tests in parallel enhances speed. Unittest executes tests sequentially. This can be slower for large test suites. It lacks built-in parallel execution, impacting speed.
Resource Utilization
Pytest efficiently manages resources. It uses less memory during test execution. Its fixtures help in optimizing resource usage. This reduces overhead and enhances performance. Unittest uses more resources. It can lead to higher memory consumption. This affects performance, especially in large projects.
Community And Support
Exploring the community and support of Pytest and Unittest can be insightful. Community plays a crucial role in adopting and efficiently using testing frameworks. Active community engagement ensures quicker problem-solving and resource availability.
Documentation
Pytest offers comprehensive documentation. Its guides are detailed and easy to follow. Beginners find it user-friendly with numerous examples. The documentation is regularly updated, addressing common issues.
Unittest documentation is thorough yet technical. It covers essential topics but may seem dense. Advanced users appreciate its detailed approach. It provides foundational understanding for testing practices.
Community Contributions
Pytest benefits from a vibrant community. Users contribute plugins and extensions. Open-source enthusiasts actively share insights. This enhances the framework’s functionality.
Unittest has a stable user base. Contributions are less frequent but reliable. Developers share best practices and code snippets. The community ensures robust testing support.
Use Cases
Comparing Pytest and Unittest reveals distinct strengths in testing Python code. Pytest offers powerful fixtures and plugins, making complex tests easier. Unittest, part of Python’s standard library, ensures compatibility and simplicity in basic testing scenarios. Each framework caters to different needs, enhancing Python testing strategies.
When you’re diving into the world of Python testing frameworks, you might find yourself choosing between Pytest and Unittest. Understanding their use cases can help you make the right decision for your project. Each framework has its strengths, and knowing when to use one over the other can streamline your testing process and boost your productivity.
When To Use Pytest
Pytest excels in scenarios where simplicity and flexibility are crucial. If you’re working on a project with complex testing requirements, Pytest’s ability to handle fixtures and parameterized testing shines. Imagine you’re developing a web application with multiple user roles and permissions. Pytest allows you to run the same test with different inputs efficiently. This feature can save you time and reduce redundant code. Pytest also integrates seamlessly with other tools and plugins, making it ideal for projects that require continuous integration. Have you ever felt bogged down by tedious setup processes? Pytest’s straightforward syntax and easy setup can alleviate that stress, making testing less of a chore.
When To Use Unit Test
Unittest is your go-to when you need a more structured approach. It is perfect for smaller projects or when you’re transitioning from other languages with similar testing frameworks like JUnit. Consider a scenario where you’re building a simple script or library. Unittest offers a clear and organized structure with classes and methods that can help keep your tests tidy and manageable.
Unittest is part of Python’s standard library, which means you don’t need to install additional packages. If you’re working in an environment where installing third-party libraries is challenging, Unittest’s availability out of the box can be a real advantage. Have you ever wondered which approach suits your style better? The choice between Pytest and Unittest often depends on your project’s scale and complexity. You might find Pytest’s flexibility appealing, or Unittest’s structure might resonate more with you. The key is to assess your specific needs and choose the framework that enhances your workflow.
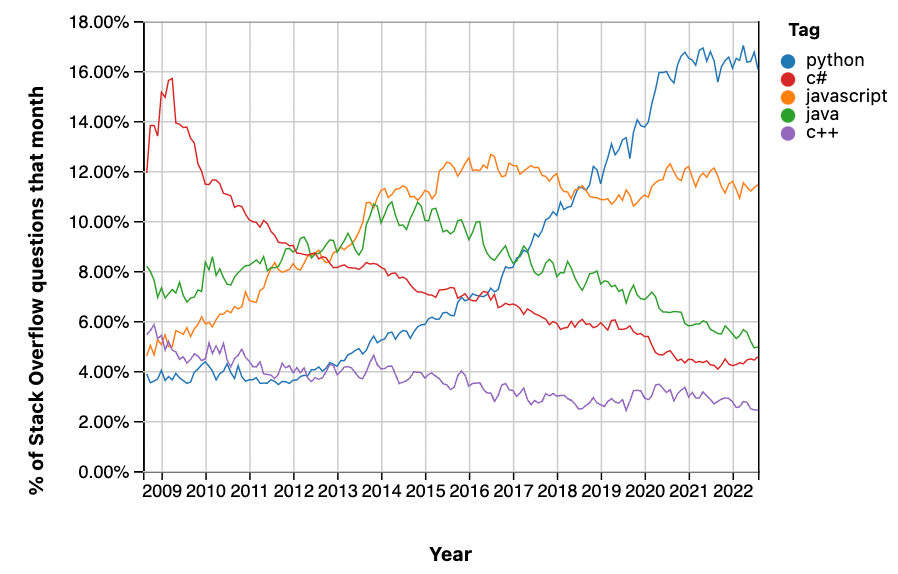
Credit: www.browserstack.com
Frequently Asked Questions
Is It Better To Use Pytest Or Unittest?
Choosing between pytest and unittest depends on your needs. Pytest offers more flexibility and features. It supports fixtures and plugins, making it ideal for large projects. Unittest is simpler, suitable for smaller projects or if you prefer a built-in library.
Both are effective for testing in Python.
Why Use Pytest-mock Instead Of Unittest Mock?
Pytest-mock offers simpler, more flexible mocking. It integrates seamlessly with pytest’s fixtures and plugins, enhancing test readability and maintainability.
Is Pytest Used For Unit Testing?
Yes, pytest is widely used for unit testing in Python. It supports fixtures, parameterized tests, and easy assertions. Pytest simplifies writing small, readable test cases. It integrates well with other tools and frameworks. Many developers prefer pytest for its flexibility and powerful features.
Why Is Pytest So Popular?
Pytest is popular for its simplicity and flexibility in testing Python code. It supports fixtures and parameterized tests, making it versatile. The tool integrates easily with other libraries and frameworks, enhancing its functionality. Its active community provides extensive documentation and plugins, ensuring robust test automation solutions.
Conclusion
Choosing between Pytest and Unittest depends on your project’s needs. Pytest offers simplicity and flexibility, perfect for complex tests. Unittest is robust and stable, ideal for basic tests. Both tools serve different purposes and complement each other well. Consider your team’s familiarity and project requirements.
Start small, experiment, and see what fits best. Testing is crucial for quality code. Make your choice wisely, and improve your testing process. Happy coding!